Your cart is currently empty!
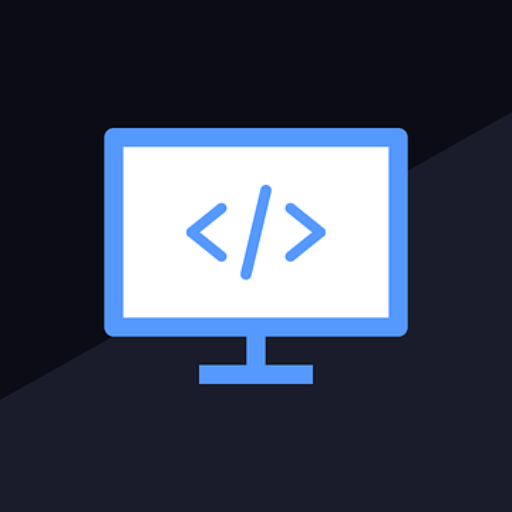
About Course
Throughout this course, we will cover fundamental concepts of Python programming, providing a solid foundation for your coding journey. Here’s a summary of the key topics and lessons you will learn:
1. Introduction to Python
- Definition: Python is a high-level, interpreted programming language known for its readability and simplicity.
- Installation: Setting up Python on your computer and running your first script.
2. Variables and Data Types
- Variables: Containers for storing data values.
- Data Types: Understanding basic data types like integers, floats, strings, and booleans.
3. Basic Operators
- Arithmetic Operators: +, -, *, /, //, %, **
- Comparison Operators: ==, !=, >, <, >=, <=
- Logical Operators: and, or, not
4. Control Structures
- Conditional Statements: if, elif, else
- Loops: for loops and while loops for iterating over sequences and repeating tasks.
5. Functions
- Definition: Reusable blocks of code that perform a specific task.
- Syntax: define keyword, parameters, return statement.
- Real-World Example: Functions for calculating the area of shapes.
6. Lists and Tuples
- Lists: Ordered, mutable collections of items.
- Tuples: Ordered, immutable collections of items.
- Common Operations: Accessing elements, slicing, adding, removing, and iterating.
7. File Handling
- Reading and Writing Files: Using
open()
,read()
,write()
,close()
, and context managers. - File Types: Handling text files, CSV files, and JSON files.
8. Error Handling
- Exceptions: Handling errors using try, except, finally, and else blocks.
- Custom Exceptions: Creating and raising custom exceptions for specific error conditions.
Key Exercises and Projects
Throughout the course, you will practise with various exercises and projects to reinforce your learning:
Exercises:
- Basic Calculations: Writing a calculator program.
- List Operations: Performing common list operations.
- Temperature Converter: Converting temperatures between Celsius and Fahrenheit.
- File Reading and Writing: Creating and reading from text files.
- Basic Exception Handling: Handling invalid inputs and division by zero.
Assignments:
- Simple Calculator: Building a calculator for basic arithmetic operations.
- Grocery List Manager: Managing a list of grocery items.
- Student Grade Calculator: Calculating and categorising student grades.
- Todo List: Creating a simple todo list application.
- Expense Tracker: Tracking expenses and calculating total spent.
- Temperature Converter: Handling custom exceptions for temperatures below absolute zero.
- Bank Account Management System: Managing bank accounts with deposits, withdrawals, and custom exceptions.
Course Content
Topic 1: Introduction to Python
-
Getting Started:
-
Exercise 1: